Debugging Code Running Under Py2exe
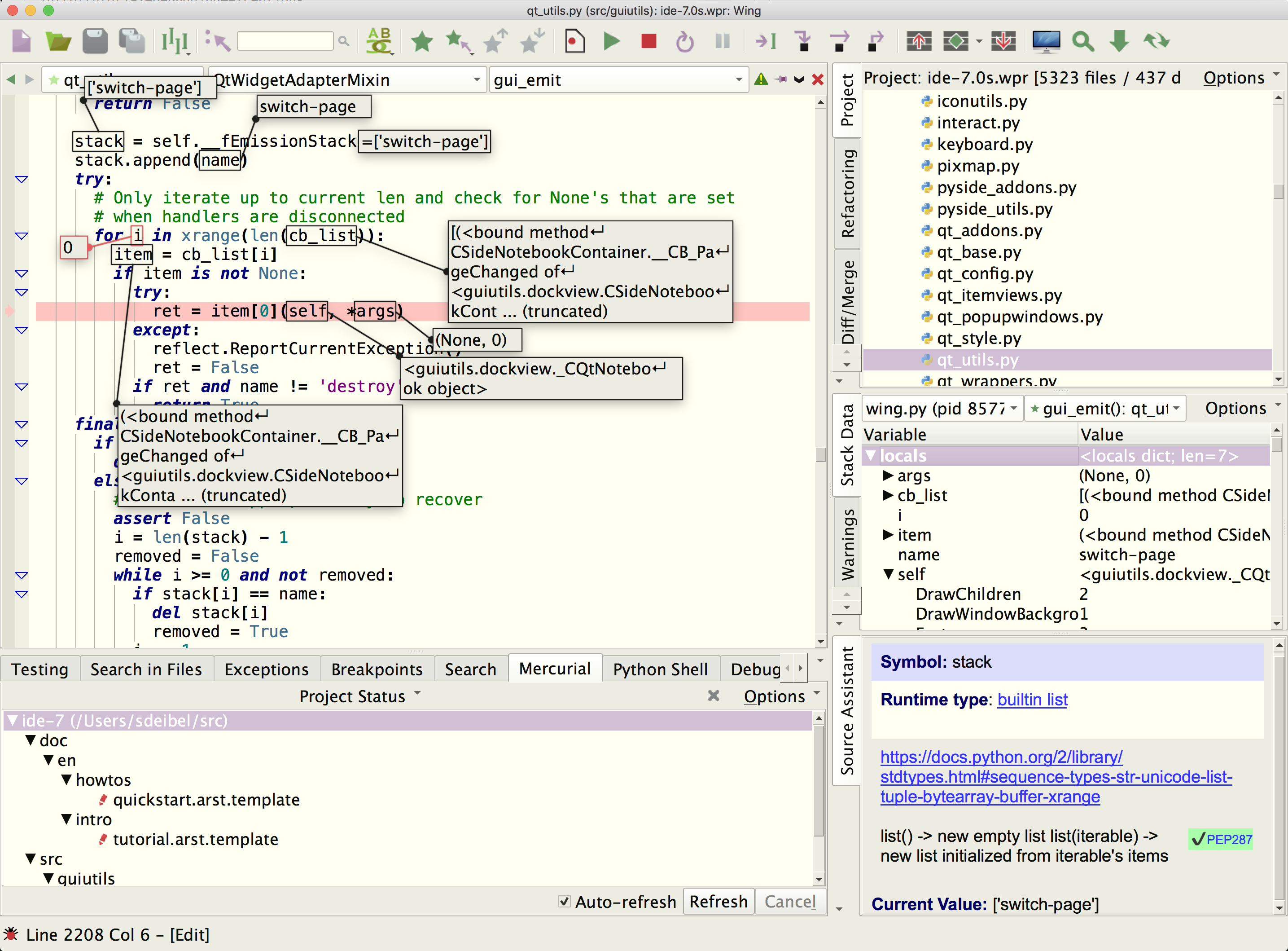
Wing Pro is a Python IDE that can be used to debug Python code running in an application packaged by py2exe. This is useful to solve a problem seen only when the code is running from the package, or so that users of the packaged application can debug Python scripts that they write for the app.
If you do not already have Wing Pro installed, download it now.
This document just describes how to configure Wing for debugging Python code running under py2exe. To get started using Wing as your Python IDE, please refer to the tutorial in Wing's Help menu or read the Quickstart Guide.
Note: This document is not maintained and was last tested in 2007.
Configuring the Debugger
To debug code running under py2exe you will need to use wingdbstub to initiate debug from outside of Wing, as described in Debugging Externally Launched Code, along with some additional configuration described below.
There are two important ways in which the environment differs when code runs under py2exe:
- When py2exe produces the *.exe, it strips out all but the modules it thinks will be needed by the application. This will remove modules needed by Wing's debugger.
- py2exe runs in a slightly modified environment and it ignores the PYTHONPATH environment.
As a result, some custom code is needed so the debugger can find and load the modules that it needs:
# Add extra environment needed by Wing's debugger
import sys
import os
extra = os.environ.get('EXTRA_PYTHONPATH')
if extra:
sys.path.extend(extra.split(os.pathsep))
print(sys.path)
# Start debugging
import wingdbstub
# Just some test code
print("Hello from py2exe")
print("frozen", repr(getattr(sys, "frozen", None)))
print("sys.path", sys.path)
print("sys.executable", sys.executable)
print("sys.prefix", sys.prefix)
print("sys.argv", sys.argv)
You will need to set the following environment variables before launching the packaged application:
EXTRA_PYTHONPATH=\Python25\Lib\site-packages\py2exe\samples\simple\dist;\Python25\lib;\Python25\dlls WINGDB_EXITONFAILURE=1
In this example, \Python25\Lib\site-packages\py2exe\samples\simple\dist contains the source for the packaged application and also the copy of wingdbstub.py used to initiate debug.
The other added path entries point at a Python installation that matches the one being used by py2exe. This is how the debugger will load missing standard library modules from outside of the py2exe package.
Setting WINGDB_EXITONFAILURE causes the debugger to print an exception and exit if it fails to load. Without this it will fail silently and continue to run without debug.
The above was tested with Python 2.5 using py2exe run with -q and -b2 options.
Related Documents
For more information see:
- py2exe home page provides downloads and documentation.
- Quickstart Guide contains additional basic information about getting started with Wing.
- Tutorial provides a gentler introduction to Wing's features.
- Wing Reference Manual documents Wing in detail.